Using Chrome Extensions
How to use Chrome extensions with Airtop
What are Chrome extensions?
Chrome extensions add additional functionality to the browser. They can automate tasks, introduce new features, or modify browser behavior.
How to use a Chrome extension
Obtaining an extension ID
Airtop only supports extensions that are published in the Google Web Store. Custom extensions are currently not supported.
To use a Chrome extension in a session, you need to provide the ID of the extension you want to use. You can find the extension ID by navigating to its page in the Chrome Web Store. The ID is the last part of the URL. For example, the extension url for the “Grammarly” is
and ID is kbfnbcaeplbcioakkpcpgfkobkghlhen
.
Using extensions in a session
Once you have the ID(s) of the extensions you want to use, you can pass them to the sessions.create
method.
Airtop will automatically install the extensions and make them available in the session.
Configuring an extension
You can access extension information and configuration pages by creating a live-view and navigating to configuration page of the extension. When the extension is installed, a new button will appear in the navigation bar, allowing you to access extension options and popup pages.
Not all extensions have options.
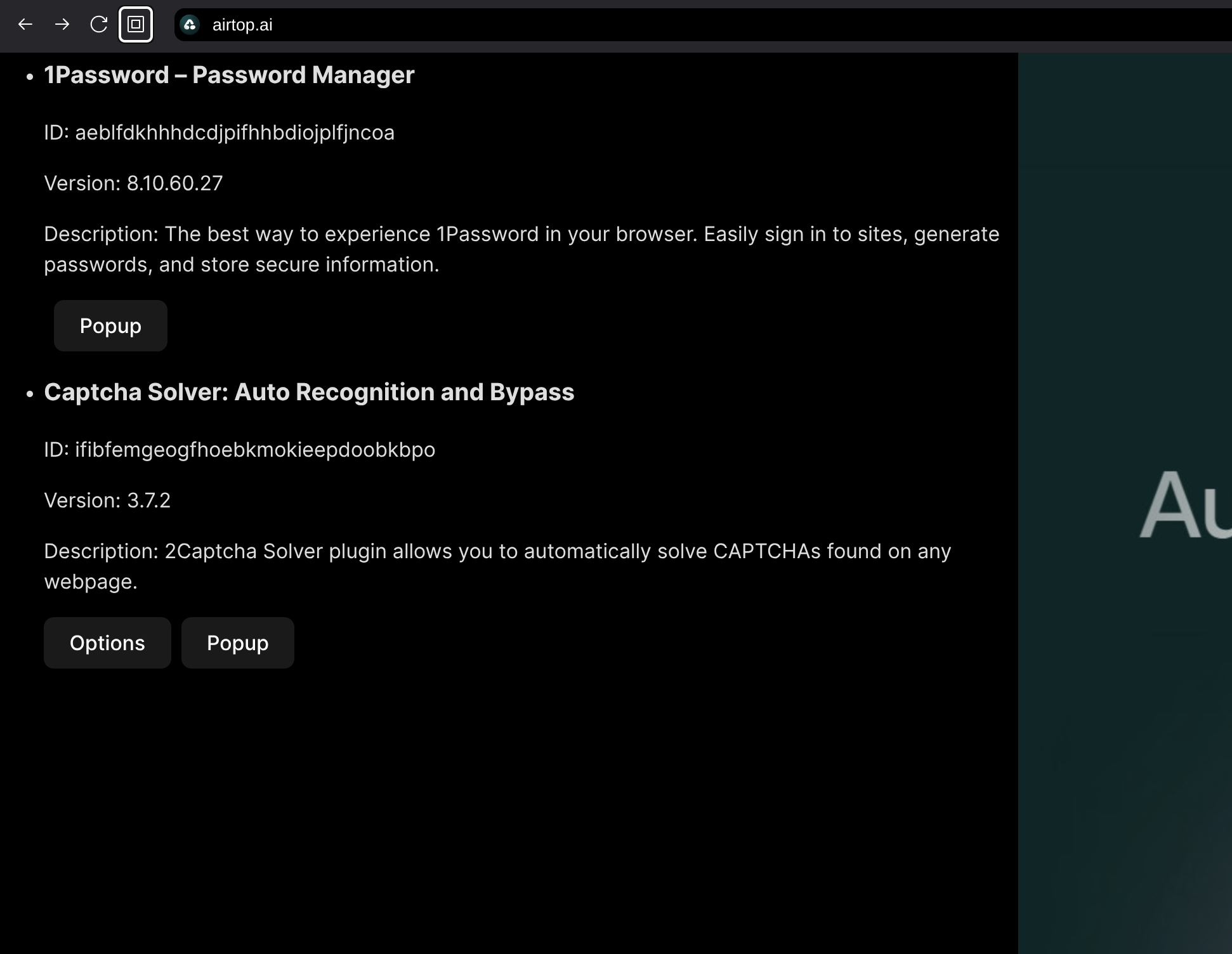
Saving extension configuration on session termination
Extension configurations are saved as part of the profile. In order to save the profile, you must call session.saveProfileOnTermination
with the name of the profile.
Extension profiles are only saved when the sesssion is terminated. You must call sessions.terminate
to ensure the profile is saved.
You can read more about profiles here.
Restoring an existing extension configuration
You can restore an existing extension configuration by specifying profile name and extension IDs in the sessions.create
method.